Implement queue using stack
The problem is opposite of this post. We are given a stack data structure with push and pop operations, the task is to implement a queue using instances of stack data structure and operations on them
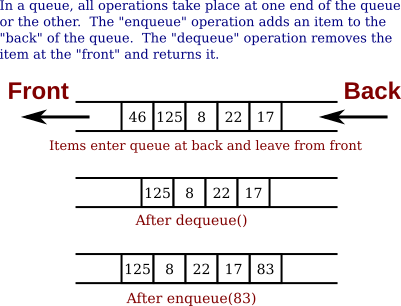
A queue can be implemented as a linked list or as an array. An efficient array
implementation is trickier than the array implementation of a stack. In the linked
list implementation, the first item of the list is at the front of the queue.
Dequeueing an item from the front of the queue is just like
popping an item off a stack. The back of the queue is at the end of the list.
Enqueueing an item involves setting a pointer in the last node of the current list
to point to a new node that contains the item.
Queues are typically used in a computer (as in real life) when only one item can be
processed at a time, but several items can be waiting for processing.
Queues are said to implement a FIFO policy: First In, First Out. Or, as it is more commonly expressed, first come, first served. Stacks, on the other hand implement a LIFO policy: Last In, First Out. The item that comes out of the stack is the last one that was put in.
IMPLEMENTATION
public
class
QueueImplement {
private
int
capacity;
int
queueArr[];
int
front =
0
;
int
rear = -
1
;
int
currentSize =
0
;
public
QueueImplement(
int
queueSize){
this
.capacity = queueSize;
queueArr =
new
int
[
this
.capacity];
}
/**
* this method adds element at the end of the queue.
* @param item
*/
public
void
enqueue(
int
item) {
if
(isQueueFull()) {
System.out.println(
"Overflow ! Unable to add element: "
+item);
}
else
{
rear++;
if
(rear == capacity-
1
){
rear =
0
;
}
queueArr[rear] = item;
currentSize++;
System.out.println(
"Element "
+ item+
" is pushed to Queue !"
);
}
}
/**
* this method removes an element from the top of the queue
*/
public
void
dequeue() {
if
(isQueueEmpty()) {
System.out.println(
"Underflow ! Unable to remove element from Queue"
);
}
else
{
front++;
if
(front == capacity-
1
){
System.out.println(
"Pop operation done ! removed: "
+queueArr[front-
1
]);
front =
0
;
}
else
{
System.out.println(
"Pop operation done ! removed: "
+queueArr[front-
1
]);
}
currentSize--;
}
}
/**
* This method checks whether the queue is full or not
* @return boolean
*/
public
boolean
isQueueFull(){
boolean
status =
false
;
if
(currentSize == capacity){
status =
true
;
}
return
status;
}
/**
* This method checks whether the queue is empty or not
* @return
*/
public
boolean
isQueueEmpty(){
boolean
status =
false
;
if
(currentSize ==
0
){
status =
true
;
}
return
status;
}
public
static
void
main(String a[]){
QueueImplement queue =
new
QueueImplement(
4
);
queue.enqueue(
4
);
queue.dequeue();
queue.enqueue(
56
);
queue.enqueue(
2
);
queue.enqueue(
67
);
queue.dequeue();
queue.dequeue();
queue.enqueue(
24
);
queue.dequeue();
queue.enqueue(
98
);
queue.enqueue(
45
);
queue.enqueue(
23
);
queue.enqueue(
435
);
}
No comments:
Post a Comment